Choosing runner#
In this tutorial, we show how to choose different “runners” to run your simulations. This is helpful if you want to change OOMMF installation you want to use. This is in particular helpful if you want to run OOMMF inside Docker, which allows us to run simulations on a “small linux machine”, which is automatically pulled from the cloud, simulations are run inside, and in the end it is destroyed automatically. This all happens in the background and requires no special assistance from the user. In order to use Docker, we need to have it installed on our machine - you can download it here: https://www.docker.com/products/docker-desktop.
For that example, we simulate a skyrmion in a sample with periodic boundary conditions.
[1]:
import oommfc as mc
import discretisedfield as df
import micromagneticmodel as mm
We define mesh in cuboid through corner points p1
and p2
, and discretisation cell size cell
. To define periodic boundary conditions, we pass an additional argument bc
. Let us assume we want the periodic boundary conditions in \(x\) and \(y\) directions.
[2]:
region = df.Region(p1=(-50e-9, -50e-9, 0), p2=(50e-9, 50e-9, 10e-9))
mesh = df.Mesh(region=region, cell=(5e-9, 5e-9, 5e-9), bc="xy")
Now, we can define the system object:
[3]:
system = mm.System(name="skyrmion")
system.energy = (
mm.Exchange(A=1.6e-11)
+ mm.DMI(D=4e-3, crystalclass="Cnv_z")
+ mm.UniaxialAnisotropy(K=0.51e6, u=(0, 0, 1))
+ mm.Zeeman(H=(0, 0, 0.2e5))
)
Ms = 1.1e6
def m_init(pos):
x, y, z = pos
if (x**2 + y**2) ** 0.5 < 10e-9:
return (0, 0, -1)
else:
return (0, 0, 1)
# create system with above geometry and initial magnetisation
system.m = df.Field(mesh, nvdim=3, value=m_init, norm=Ms)
Now, we can define the runner object. There are three main runners you can use:
Tcl runner: if we want to point ubermag to the particular
oommf.tcl
fileExe runner: if we have OOMMF executable
Docker runner: if we want to run simulations inside Docker container
[4]:
tcl_runner = mc.oommf.TclOOMMFRunner(oommf_tcl="path/to/my/oommf.tcl")
couldn't read file "path/to/my/oommf.tcl": no such file or directory
[5]:
exe_runner = mc.oommf.ExeOOMMFRunner(oommf_exe="oommf")
[6]:
docker_runner = mc.oommf.DockerOOMMFRunner(image="ubermag/oommf")
IMPORTANT: On Windows, if OOMMF does not support some energy terms, choosing runner happens automatically in the background and requires no assistance from the user. However, you can still be explicit and tell ubermag how you want to run the simulation.
Now, when we drive the system, we can pass the runner to the drive
method:
[7]:
md = mc.MinDriver()
md.drive(system, runner=exe_runner)
# Plot relaxed configuration: vectors in z-plane
system.m.sel("z").z.mpl()
Running OOMMF (ExeOOMMFRunner)[2023/10/23 16:07]... (0.5 s)
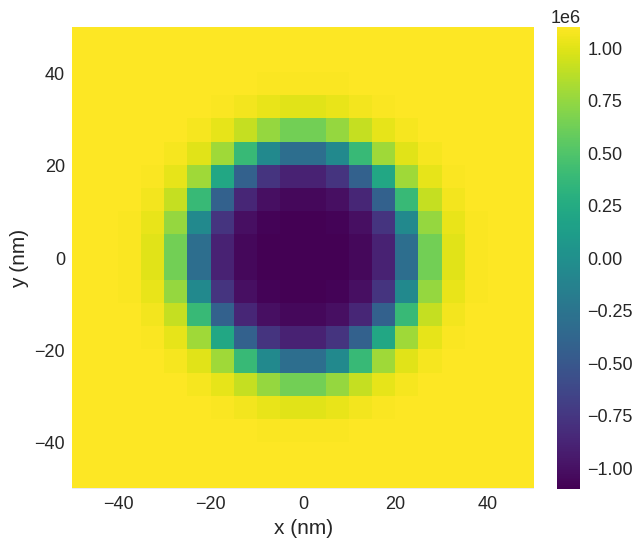
The first time we run the simulation, it is going to take some time for docker to pull an image from the cloud, but after that, the image will be known by docker, so there will be no delays for any further runs.