Fixed subregions#
It is sometimes necessary to “fix” specific regions of the mesh to ensure they do not change during driving. In order to do that, the first step is to create a mesh and specify subregions we want to keep fixed.
As an example, let us simulate a simple one-dimensional sample and define subregions in such a way that the first and the last discretisation cell remain fixed.
[1]:
import oommfc as mc
import discretisedfield as df
import micromagneticmodel as mm
region = df.Region(p1=(-30e-9, 0, 0), p2=(30e-9, 3e-9, 3e-9))
cell = (3e-9, 3e-9, 3e-9)
subregions = {'fixed1': df.Region(p1=(-30e-9, 0, 0), p2=(-27e-9, 3e-9, 3e-9)),
'free': df.Region(p1=(-27e-9, 0, 0), p2=(27e-9, 3e-9, 3e-9)),
'fixed2': df.Region(p1=(27e-9, 0, 0), p2=(30e-9, 3e-9, 3e-9))}
mesh = df.Mesh(region=region, cell=cell, subregions=subregions)
[2]:
mesh.mpl.subregions(box_aspect=(10, 3, 3), figsize=(12, 12))
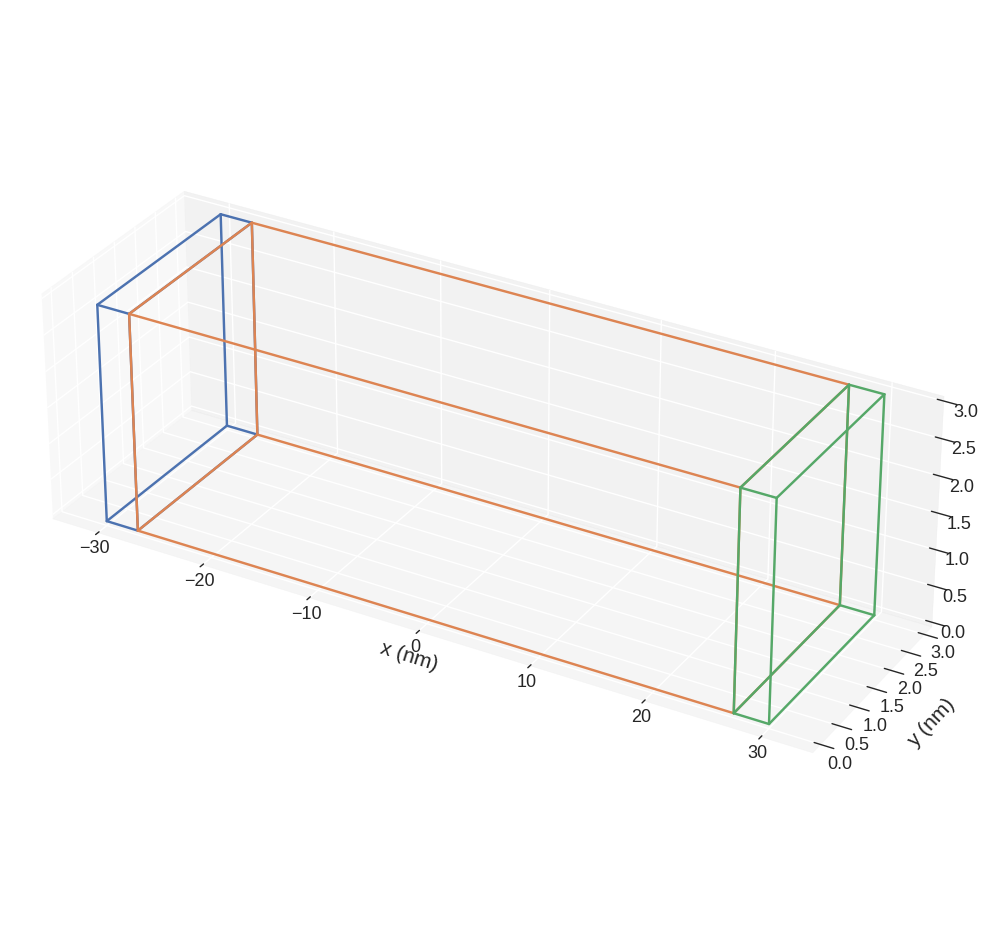
Now, let us define a system whose energy equation consists of only exchange energy:
[3]:
system = mm.System(name='fixed_spins')
system.energy = mm.Exchange(A=1e-12)
We are going to initialise the magnetisation in fixed1
region to be \((0, 0, 1)\) and in fixed2
region \((0, 0, -1)\). In the free
region, we are going to choose \((1, 0, 0)\) for initial magnetisation.
[4]:
m = {'fixed1': (0, 0, 1), 'fixed2': (0, 0, -1), 'free': (1, 0, 0)}
system.m = df.Field(mesh, nvdim=3, value=m, norm=1e6)
The magnetisation is now:
[5]:
system.m.sel('y').mpl(
figsize=(12, 2),
scalar_kw={"colorbar_label":"$m_y$"},
)
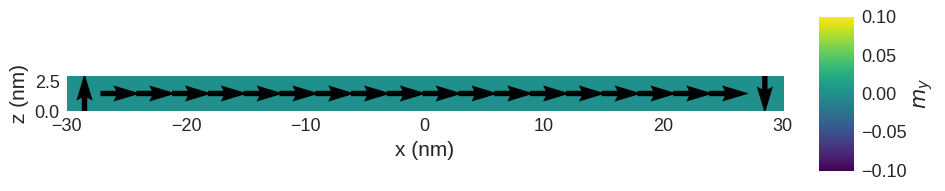
Finally, we can drive the system. In this case, we are going to choose MinDriver
. When we call drive
method, we have to pass fixed_subregions
argument, which is a list of subregions names we want to keep fixed duriing relaxation.
[6]:
md = mc.MinDriver()
md.drive(system, fixed_subregions=['fixed1', 'fixed2'])
Running OOMMF (ExeOOMMFRunner)[2023/10/23 16:08]... (0.4 s)
The relaxed magnetisation is:
[7]:
system.m.sel('y').mpl(
figsize=(12, 2),
scalar_kw={"colorbar_label":"$m_y$"},
)
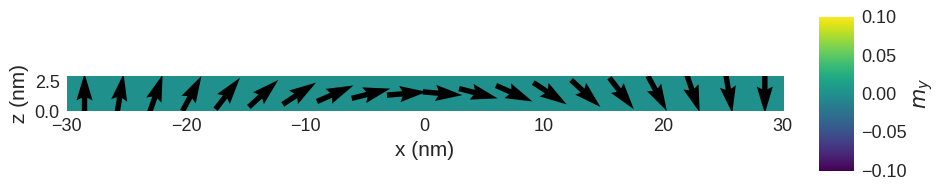
From the resulting magnetisation field, we can see that the first and the last spin have remained the same as in the initial magnetisation and a Neel domain wall has formed in between due to the exchange energy.